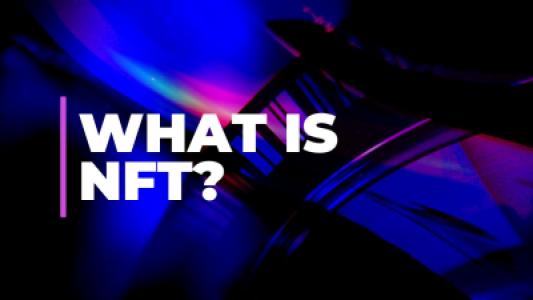
Web3
When you start learning React.js, one of the first concepts you’ll come across is JSX (JavaScript XML). At first glance, JSX might seem unusual—almost like embedding HTML inside JavaScript. However, it’s a fundamental feature of React that makes UI development more intuitive and efficient.
In this blog, we’ll explore:
By the end of this guide, you'll have a solid understanding of JSX and how to use it effectively to build dynamic user interfaces in React.js.
JSX stands for JavaScript XML. It’s a syntax extension of JavaScript that allows developers to write HTML-like code inside JavaScript files. Instead of keeping markup and logic separate, JSX enables you to combine them, making it easier to build dynamic user interfaces in React.js.
JSX is an extension of the JavaScript language based on ES6. It gets translated into regular JavaScript at runtime, ensuring compatibility with browsers that do not natively understand JSX.
Although JSX resembles HTML, it isn’t valid JavaScript or HTML. Instead, it’s a syntactic sugar that React uses to make writing components more intuitive. Behind the scenes, JSX is compiled into standard JavaScript using the React.createElement function.
Here’s a simple example of JSX:
const element = <h1>Hello, world!</h1>;
JSX simplifies the process of writing HTML inside React applications, making the code more readable, maintainable, and efficient. It also helps in:
✅ Enhancing code readability – JSX provides a clear structure, making it easier to understand the UI logic.
✅ Boosting development speed – Developers can write components faster without worrying about manually creating DOM elements.
✅ Leveraging JavaScript’s power – JSX allows you to use JavaScript expressions within curly braces {} inside your markup.
const myElement = React.createElement('h1', { style: { color: "green" } }, 'I do not use JSX!');
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(myElement);
const myElement = <h1 style={{ color: "green" }}>I Love JSX!</h1>;
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(myElement);
Although JSX looks like HTML, it behaves differently. Understanding these differences is crucial for writing efficient React code. Let’s explore some key distinctions between JSX and regular JavaScript.
isn’t directly understood by browsers. Instead, React uses Babel to convert JSX into standard JavaScript.
Example JSX code:
const element = <h1>Hello, world!</h1>;
What it transforms into:
const element = React.createElement('h1', null, 'Hello, world!');
Essentially, when you write JSX, you're creating JavaScript objects that represent DOM elements.
Unlike regular HTML, JSX follows JavaScript’s camelCase naming convention for attributes.
Common attribute differences:
Example JSX button:
const button = <button onClick={handleClick}>Click Me</button>;
This ensures consistency with JavaScript’s syntax rules.
One of JSX’s biggest advantages is embedding JavaScript expressions inside curly braces {}. This allows you to dynamically update UI elements.
Conditional Rendering in JSX
const isLoggedIn = true;
const greeting = (
<div>{isLoggedIn ? <h1>Welcome back!</h1> : <h1>Please sign in.</h1>}</div>);
Without JSX, you'd need to manually manipulate the DOM, making your code more complex and error-prone.
JSX must be wrapped inside a single parent element; otherwise, React will throw an error.
Incorrect JSX (will cause an error):
const element = (
<h1>Hello</h1>
<p>Welcome to the website!</p>
);
Correct JSX using a wrapper:
const element = (
<div><h1>Hello</h1><p>Welcome to the website!</p></div>);
Alternative: Use React Fragments to avoid unnecessary
const element = (<><h1>Hello</h1> <p>Welcome to the website!</p></>);
This keeps your JSX cleaner and prevents unnecessary nesting in the DOM.
Now that you understand what JSX is and how it differs from regular JavaScript, let’s explore some best practices to help you write clean, maintainable, and efficient JSX code in your React applications.
One of the key advantages of JSX is that it encourages component-based architecture. To make the most out of this, break down your UI into small, reusable components. This makes your code more manageable and promotes reusability.
Why?
Always use meaningful, descriptive names for your components and variables. This makes your code easier to read and helps other developers understand its purpose at a glance.
Example:
Instead of naming a button component simply Button, consider naming it SubmitButton if it’s specifically used to submit a form.
const SubmitButton = () => <button type="submit">Submit</button>;
This enhances clarity and prevents confusion as your codebase grows.
JSX makes it easy to conditionally render components using ternary operators, &&, or if statements. However, it's important to keep your conditional logic simple and readable.
Good Practice:
Example:
const isLoggedIn = true;
const greeting = (<div>{isLoggedIn ? <h1>Welcome back!</h1> : <h1>Please sign in.</h1>}</div>);
Tip:
For complex logic, move it outside the JSX and then return the result inside your component.
Excessive nesting of JSX elements can make your code difficult to read and maintain. If your JSX starts becoming too deep, it's a clear sign that you should consider breaking it down into smaller, more manageable components.
Why Avoid Excessive Nesting?
Example of Excessive Nesting
const element = (<div><div><div><h1>Welcome</h1><p>Enjoy your stay!</p></div> </div></div>);
Better Approach
Consider creating separate components for each section.
const WelcomeMessage = () => <h1>Welcome</h1>;
const StayMessage = () => <p>Enjoy your stay!</p>;
const element = (
<div>
<WelcomeMessage />
<StayMessage />
</div>
);
By following these best practices, you can write clean, efficient, and maintainable JSX in your React projects. Keep your components small and reusable, use descriptive names, handle conditional rendering carefully, and avoid unnecessary nesting.
While working with JavaScript we typically have a HTML file, a CSS file, and a JavaScript file, right? Simple. But while working with React, we use HTML (JSX) and JavaScript in the same file.
To add style to our components, we can either use inline CSS or external CSS.
Let's first see how inline CSS works in React.
JSX represents objects, that is key-value pairs – like a property name and its value. Always write the value in " " as we do in objects.
So when we're adding inline styles to JSX elements, we must add { } for object representation (outer { } is the convention).
const App = () => {
return (
<>
<h1 style={ { color: "Red" } }>Hello World!</h1>
<p style={ { fontSize: "20px" } }>Tanishka here!</p>
</>
);
}
Here, if you look at style in isolation, you'll see it does look like an object:
{
color: "Red"
}
Basically, use { } to add styles, use key-value pairs, and always write the value in " ".
Now, let's see how to use an external CSS file.
We simply create a .css file and import it in our .jsx file like this:
import './style.css'
/*
Your code here
*/
We might need to use plain JavaScript to add logic to the program rendering components. And to add plain JavaScript code in JSX, we need to write it inside curly brackets.
However, whatever we write inside curly brackets must be an expression. Statements are not allowed.
const App = () => {
return (
<>
<h1>My name is {const name = "Tanishka"}</h1>
</>
);
}
The above code is invalid.
Now, you might think {const name = "Tanishka"}
is also JavaScript. How is this code block not valid? Because it is an initialization of name that is a statement and we cannot write statements inside curly brackets.
We can make a few changes in the above code block and it will be valid:
const App = () => {
return (
const name = "Tanishka";
<>
<h1>My name is {name}</h1>
</>
);
}
We initialized value of name outside the return block and just used the included varibale name inside curly brackets, where name has the assigned value of "Tanishka". So now it is an expression and is valid.
Now that we know how to write code in React, let's learn what to write – that is, React's basic concepts.
When you're working with React, you often need to return more than just a simple element. Sometimes, your component needs to render a more complex structure that includes multiple elements or components. Here's how you can handle this in an easy-to-understand way:
In React, every JSX return must have one parent element. This means you can't return multiple elements side by side unless they are wrapped in a single parent element (like a
, a
, or a React Fragment).
For example:
const MyComponent = () => {
return (
<div>
<h1>Title</h1>
<p>Here is some content.</p>
</div>
);
};
In this example, both the and tags are wrapped in a. This satisfies the rule that JSX must return one parent element.
Sometimes, you don’t want to add unnecessary wrapper elements to your JSX, such as a or a. In those cases, you can use React Fragments. Fragments let you group a list of children without adding extra nodes to the DOM.
Here's how you can use a fragment:
const MyComponent = () => {
return (
<>
<h1>Title</h1>
<p>Here is some content.</p>
</>
);
};
With a fragment, you're still returning multiple elements, but React doesn't add a parent element like, keeping your DOM structure clean.
Sometimes you need to render different JSX elements based on a condition (like checking if a user is logged in or not). You can use JavaScript conditional logic inside JSX to do this.
Example with a ternary operator:
const MyComponent = ({ isLoggedIn }) => {
return (
<>
{isLoggedIn ? (
<h1>Welcome back!</h1>
) : (
<h1>Please sign in.</h1>
)}
</>
);
};
This component checks the isLoggedIn prop, and if it's true, it displays a welcome message; otherwise, it asks the user to sign in.
Sometimes you may want to return multiple elements without wrapping them in a single parent element. You can return an array of JSX elements, but keep in mind that the elements in the array need to have unique keys if they are part of a list.
Here’s how to return an array:
const MyComponent = () => {
return [
<h1 key="1">Title</h1>,
<p key="2">This is a paragraph.</p>,
<button key="3">Click me!</button>
];
};
In this case, the component returns an array of JSX elements. Each element has a unique key attribute, which is required when returning lists in React.
You can also split your component logic into smaller functions to return different sections of JSX. This keeps your code organized and readable.
For example, breaking down the rendering logic into smaller parts:
const Header = () => {
return <h1>Welcome to My Website</h1>;
};
const Content = () => {
return <p>This is the main content of the page.</p>;
};
const MyComponent = () => {
return (
<>
<Header />
<Content />
</>
);
};
};
Here, the MyComponent function returns a complex JSX structure by reusing smaller components (Header and Content).
By understanding and applying these techniques, you'll be able to return and manage complex JSX in your React components with ease.
© themes-park | All Rights Reserved