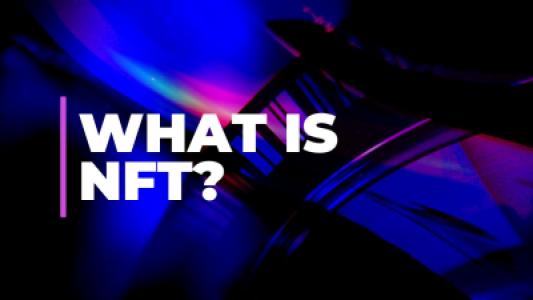
Web3
State management is a core concept in React development. As applications grow in complexity, handling state effectively becomes more challenging. From small apps to large-scale enterprise-level solutions, choosing the right state management strategy can have a significant impact on performance, maintainability, and scalability.
Previous blog we covered React parent & child state management and props passing. In this blog post, we'll dive deep into React state management, explore the most popular tools, and help you choose the best approach based on the scale of your application. Whether you're using React Context API, Redux, or emerging tools like Recoil, we've got you covered.
Before we dive into the tools, it's essential to understand the difference between local and global state in React.
Local State: Refers to state that’s managed within a single component using useState
. It’s typically used for simple UI states, like toggling a button, form input, etc.
Global State: Refers to state that needs to be shared across multiple components. It’s useful when different parts of your app need access to the same state data (e.g., user authentication status, cart items, etc.).
Understanding the difference will help you decide whether you need to introduce state management solutions beyond React's built-in state.
useState
and useReducer
For many small to medium-sized applications, React's built-in state management hooks, such as useState
and useReducer
, can be sufficient.
useState: Ideal for local component state management. Simple and easy to use.
Example:
const [count, setCount] = useState(0);
return <button onClick={() => setCount(count + 1)}>{count}</button>;
useReducer: Provides a more complex state management solution, ideal for handling more intricate state logic, especially in larger components or applications with multiple state transitions.
Example:
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
const [state, dispatch] = useReducer(reducer, initialState);
While these hooks are sufficient for local state management, when your app grows in complexity, you’ll need to manage state globally. This is where tools like React Context API, Redux, and others come into play.
The Context API is a powerful built-in solution for managing global state in React. It allows you to avoid prop-drilling by providing a way to share state across your component tree without manually passing props.
However, the Context API is not always the best solution for large-scale state management due to potential performance issues with re-renders.
When to Use Context API:
Simple apps with minimal state management needs.
Sharing state between a few components that are not deeply nested.
Lightweight global state like theme settings, user authentication status, etc.
Example:
const UserContext = React.createContext();
function App() {
const [user, setUser] = useState(null);
return (
<UserContext.Provider value={{ user, setUser }}>
<Header />
<UserProfile />
</UserContext.Provider>
);
}
function Header() {
const { user } = useContext(UserContext);
return <h1>{user ? `Hello, ${user.name}` : 'Welcome!'}</h1>;
}
Problem:
Performance Issues: Every time a context value changes, all consumers re-render. For large apps, this can lead to performance bottlenecks.
Limited Features: Unlike more dedicated state management solutions, Context doesn’t offer advanced features like middleware or time-travel debugging.
For larger applications with complex state and frequent interactions, Redux is the go-to solution for state management. Redux is a predictable state container for JavaScript apps and integrates well with React.
Why Use Redux?
Predictable State: Redux uses a central store, and state updates happen through dispatched actions. This makes the flow of data predictable and debuggable.
Middleware Support: Redux supports middleware like Redux Thunk and Redux Saga for handling asynchronous operations.
Developer Tools: Redux provides excellent development tools like time-travel debugging, action logging, and more.
When to Use Redux:
Large-scale applications with complex state logic.
Applications with many different pieces of state that need to be updated simultaneously.
Projects requiring advanced debugging tools or middleware support.
Example:
// Reducer
const userReducer = (state = {}, action) => {
switch (action.type) {
case 'SET_USER':
return { ...state, user: action.payload };
default:
return state;
}
};
// Store
const store = createStore(userReducer);
// Action
const setUser = (user) => ({ type: 'SET_USER', payload: user });
// Component
const UserProfile = () => {
const user = useSelector(state => state.user);
const dispatch = useDispatch();
useEffect(() => {
dispatch(setUser({ name: 'John Doe' }));
}, [dispatch]);
return <div>{user ? user.name : 'Loading...'}</div>;
};
Challenges with Redux:
Boilerplate Code: Redux can require a lot of boilerplate code, especially for simple use cases.
Learning Curve: While powerful, Redux can be more difficult to learn for beginners compared to other state management solutions.
Recoil is a newer state management library from Facebook that is gaining popularity due to its simplicity and powerful features. It works seamlessly with React and offers a more modern and flexible approach compared to Redux.
Key Features of Recoil:
Atoms and Selectors: Atoms represent state, and selectors allow you to compute derived state, making it easy to manage complex state logic.
Better Performance: Unlike Context API, Recoil minimizes unnecessary re-renders, making it more performant.
Built for React: Recoil is designed to take full advantage of React’s concurrent rendering.
When to Use Recoil:
Medium to large applications where state management needs to be simple and efficient.
Developers looking for a more modern alternative to Redux with less boilerplate code.
Example:
import { atom, useRecoilState } from 'recoil';
const userState = atom({
key: 'userState',
default: null,
});
function UserProfile() {
const [user, setUser] = useRecoilState(userState);
return (
<div>
{user ? <h1>Hello, {user.name}</h1> : <h1>Loading...</h1>}
</div>
);
}
When deciding between Context API, Redux, Recoil, or other state management tools, consider the following:
Context API is great for small apps or simple global state.
Redux is the best choice for large, complex applications with lots of state changes and side effects.
Recoil is a modern alternative, designed to be simpler and more performant for React developers who need more flexibility than Context API provides but don’t need all of Redux’s features.
Choosing the right React state management solution for your app depends on the complexity of your project and the type of state you need to manage. From React's built-in hooks for simple apps to Redux and Recoil for more complex applications, there’s no one-size-fits-all solution. Carefully evaluate your app’s needs and choose the state management tool that provides the right balance of simplicity, performance, and scalability.
Hope this blog helps you to be better at choosing the best React state management approach for your app, Happy coding.
© themes-park | All Rights Reserved