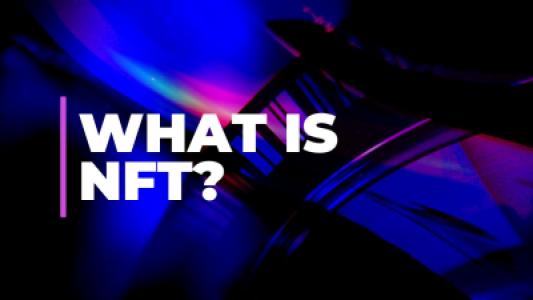
Web3
React state management is at the core of building dynamic and interactive web applications. Whether you're new to React or looking to refine your skills, understanding how state flows between parent and child components and how to pass props effectively is essential for building maintainable apps. In this guide, we’ll dive into how React handles state, props, and the communication between components.
State management in React refers to how you manage and share data across your components. React components can have their own state, and managing this state efficiently is crucial for building complex, interactive UIs.
React provides several ways to manage state, from local state within a component to global state that is shared across the application. But today, we'll focus on a fundamental concept: parent-child state management and how props are used to handle data flow between components.
In React, state refers to data that can change over time, affecting how a component renders. Unlike props, which are passed from parent to child, state is local to the component, meaning it can be changed by the component itself.
The useState
hook is the most commonly used hook for handling state in functional components.
const [state, setState] = useState(initialState);
Here, state
is the value, and setState
is the function used to update it.
In React, the parent component holds the state, and the child component can access and modify it via props. This is the most common pattern for state management.
In React, the parent component manages the state, while the child component receives it as props. Although the child can read the prop, it cannot modify it directly. Instead, the parent typically provides a function that the child can call to update the state.
Example:
function ParentComponent() {
const [count, setCount] = useState(0);
return (
<div>
<h1>Count: {count}</h1>
<ChildComponent count={count} setCount={setCount} />
</div>
);
}
function ChildComponent({ count, setCount }) {
return (
<div>
<button onClick={() => setCount(count + 1)}>Increment</button>
<p>Child sees count: {count}</p>
</div>
);
}
Here, the ParentComponent
holds the state (count
) and passes it to the ChildComponent
as a prop. The child uses setCount
to update the parent's state.
When the child component needs to change the parent's state, it uses a function passed as a prop from the parent, a concept called lifting state up.
Example:
function ParentComponent() {
const [message, setMessage] = useState('');
const handleChangeMessage = (newMessage) => {
setMessage(newMessage);
};
return (
<div>
<h1>{message}</h1>
<ChildComponent onMessageChange={handleChangeMessage} />
</div>
);
}
function ChildComponent({ onMessageChange }) {
return (
<input
type="text"
onChange={(e) => onMessageChange(e.target.value)}
placeholder="Enter message"
/>
);
}
Here, the child calls the onMessageChange
function, which updates the parent's state.
In React, props are used to pass data from parent components to child components. Props are read-only and cannot be modified by the child component directly. Instead, they serve as a way to communicate data from the parent to child components in a top-down fashion.
Props allow for passing dynamic data into a component. They make components reusable and flexible. Props can be anything—strings, numbers, arrays, objects, or even functions.
Example:
function ParentComponent() {
const title = 'Welcome to React!';
return <ChildComponent title={title} />;
}
function ChildComponent({ title }) {
return <h1>{title}</h1>;
}
In this example, the parent passes a string (title
) to the child component. The child accesses it through destructuring.
Props help make components highly reusable. By accepting different props, components can be easily customized for different use cases.
Example of a Reusable Button Component:
function Button({ label, onClick }) {
return <button onClick={onClick}>{label}</button>;
}
function ParentComponent() {
const handleClick = () => alert('Button clicked!');
return <Button label="Click Me" onClick={handleClick} />;
}
This button is completely reusable—by passing different label
and onClick
props, we can reuse it in various places.
To ensure the right data types are passed to components, prop-types can be used for type-checking.
import PropTypes from 'prop-types';
function Button({ label }) {
return <button>{label}</button>;
}
Button.propTypes = {
label: PropTypes.string.isRequired,
};
This ensures that the label
prop is always a string and required. Prop types are a very useful tool for catching bugs early in development and make development easier to understand.
While parent-child state management works for smaller applications, larger applications often require a more scalable solution. React's Context API allows you to manage global state in your app and share it across components without needing to pass props down manually at every level.
For more complex state management, libraries like Redux or Recoil can be used to manage state globally, making it easier to handle larger apps with complex data flows.
Effective state management is crucial to building scalable and maintainable React applications. Understanding how state flows between parent and child components, managing props, and using advanced techniques like lifting state up will empower you to create cleaner, more efficient code.
For large applications, consider using React Context API, Redux, or Recoil to handle more complex state management needs. With these tools, you can build robust applications that are easy to maintain and scale. We will be going to cover these on the next part.
Hope these helps you on your development process, Happy coding.
© themes-park | All Rights Reserved