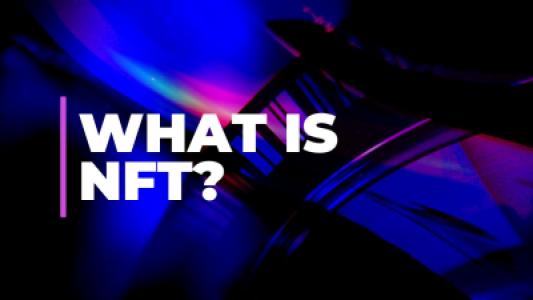
Web3
Laravel has taken the web development world by storm—and for good reason. With its elegant syntax and powerful tools, Laravel helps developers build fantastic web applications quickly and efficiently. But even with such a great framework, you can run into performance issues if you don't pay attention to certain best practices.
In this blog, we’ll go over 10 simple yet highly effective tips to help you supercharge the performance of your Laravel web apps. Whether you’re dealing with a small project or a massive application, these tips will help your app run faster and more efficiently.
One of the most common causes of slow Laravel apps is inefficient database queries.
Eager Loading: Instead of loading relationships one by one (which can result in many queries), use eager loading to grab all necessary data in a single query.
Example:
$users = User::with('posts')->get();
Query Caching: Laravel provides built-in support for caching your queries, which means your app won’t have to hit the database every time it needs the same data.
Example:
$users = Cache::remember('users', 60, function () {
return User::all();
});
By being mindful of your queries and caching, you’ll notice a significant performance improvement.
Rendering views on each page load can be a slow process, especially if you have complex Blade templates. Use Laravel’s view cache to speed things up.
You can cache views by running:
php artisan view:cache
This command will precompile your Blade views and store them, making them much faster to load.
Laravel’s caching system is a game-changer when it comes to optimizing performance. Whether you’re using Redis, Memcached, or the database, caching allows you to store and quickly retrieve commonly used data.
Tip: Cache API responses or database query results that don’t change often.
$posts = Cache::remember('posts', 60, function () {
return Post::all();
});
Caching helps your app avoid redundant work, which means faster response times.
Clean, organized routes make a big difference in performance. Here's how you can improve your routing setup:
Group Routes: For routes that share the same middleware or behavior, grouping them can reduce repetition.
Route::middleware('auth')->group(function () {
Route::get('profile', 'UserController@profile');
Route::get('dashboard', 'UserController@dashboard');
});
php artisan route:cache
Time-consuming tasks like sending emails or processing images can slow down your app. Instead of running these tasks during a request, offload them to Laravel’s job queues.
This means your users won’t experience delays while your app handles background tasks. It keeps the app responsive, even when working with large data.
Example:
dispatch(new SendEmailJob($user));
If your app has a large number of classes, it’s essential to optimize Composer’s autoloader. This will reduce the time it takes to load your classes and speed up the app.
Run the following commands to optimize autoloading:
composer install --optimize-autoloader
composer dump-autoload --optimize
Indexes can drastically speed up your database queries, especially for large tables. Always make sure to index the columns you frequently query.
Example:
Schema::table('users', function (Blueprint $table) {
$table->index('email'); // Index email column
});
When serving your Laravel app, make sure to enable HTTP/2 and HTTPS. HTTP/2 allows for faster loading speeds, especially on mobile devices, by allowing multiple requests over a single connection. Plus, Google loves HTTPS, and it’ll help with your SEO rankings.
Tip: You can easily enable HTTPS by obtaining an SSL certificate and forcing HTTPS in Laravel’s middleware.
Large JavaScript and CSS files can slow down your app, especially when your users are on slower connections. Use Laravel Mix to minify and combine your assets into smaller, faster files.
mix.js('resources/js/app.js', 'public/js')
.sass('resources/sass/app.scss', 'public/css')
.version();
This reduces the number of HTTP requests and speeds up your page load time.
If your app relies heavily on queues, consider using Laravel Horizon. It provides an easy-to-use dashboard to monitor and manage your queues in real time. Horizon helps you keep track of failed jobs, queue performance, and more.
composer require laravel/horizon
php artisan horizon:install
This tool is invaluable for scaling large applications that rely on queues.
Performance should always be top of mind when building Laravel applications. By following these 10 simple yet powerful tips, you can optimize your app and ensure it runs faster, even under heavy traffic. From query optimization to offloading tasks to queues and enabling caching, these practices will make a significant difference.
Hope these tips will help you creating more efficient apps, Happy coding.
© themes-park | All Rights Reserved